Scala Median function
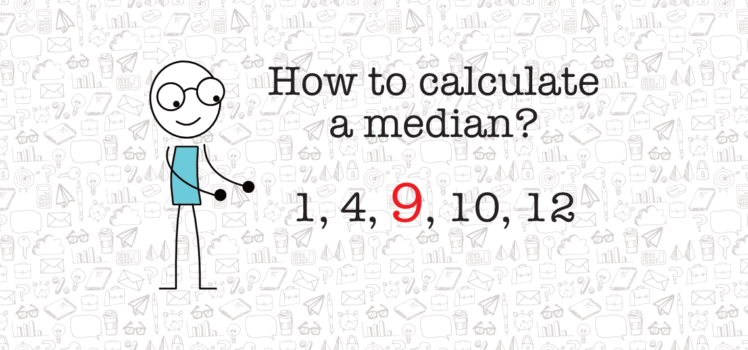
Have you ever heard about a median? I’m talking about that thing from mathematician statistics, which represents a “middle” value of a data set. If you are reading this, I bet you hear about it. Moreover you want to know how to implement a median function in Scala. So I’ll show you how it can be done.
Theory
Let’s start from a theory introduction. In case if you have a theoretical background about median, skip this section and go ahead.
Here is a sample data sets of integer values:
A: [3, 5, 10, 11, 19] B: [1, 5, 14, 16, 17, 20]
Notice 2 important things:
- A & B are sorted
- A & B have odd and even quantity of elements
Let’s calculate medians for A & B sequences:
Median of A is 10
Median of B is (14 + 16) / 2 = 15
That’s it. Nothing complex, but there are several things which I have had to point out before the implementation.
Implementation
val aSet = Seq(3, 5, 10, 11, 19) val bSet = Seq(1, 5, 14, 16, 17, 20) def medianCalculator(seq: Seq[Int]): Int = { //In order if you are not sure that 'seq' is sorted val sortedSeq = seq.sortWith(_ < _) if (seq.size % 2 == 1) sortedSeq(sortedSeq.size / 2) else { val (up, down) = sortedSeq.splitAt(seq.size / 2) (up.last + down.head) / 2 } } medianCalculator(aSet) //10 medianCalculator(bSet) //15
Run this code and you will get the results which correspond to median values of data sets. By the way, if you have improved versions of median calculator function, please leave it in comments.