Scala: Convert List[B[A]] into List[A]
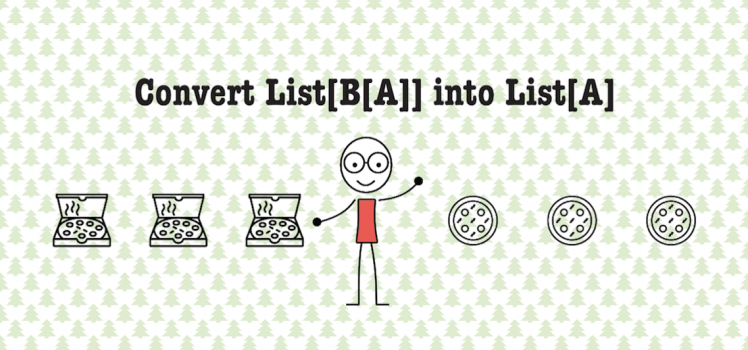
Hey Scala squad! In this post I’m going to show some ways of extracting values from list or sequence of boxed elements. It’s a pretty common case when you have a Seq[B[A]]
, but instead of it you need to get Seq[A]
. To be more precise it may be Seq[Try[String]]
or List[Option[Int]]
.
It’s not a big secret that Scala has a lot of powerful functions for collection manipulations. But in what order it’s better to apply them to achieve the desired result? I prepared multiple examples of how this may be solved. If you have better ideas, just put them in the comments.
In order to show how to flatten list of Try
or Option
or whatever box type we need to introduce some sequence example.
val listOfTries: Seq[Try[String]] = Seq( Success("Yes"), Success("Ok"), Failure(new Exception("No")), Success("Deal"))
So the first method to extract String
s from listOfTries
is to filter all of the elements by applying isSuccess
method and then map each of success elements to its internal string value.
val listOfStrings = listOfTries.filter(_.isSuccess).map(_.get)
It’s ok, as for me, but there may be even more concise solution:
val listOfStrings = listOfTries.collect { case Success(str) => str }
Since collect
accepts PartialFunction
, we just iterating through all of the elements, match them to Success
and finally retrieve their values.
Summary
The same approach may be used for processing of sequences with Option
elements. But I’m still curious about your thoughts regarding the solutions which I illustrated above. You are more than welcome to comment this short article 🙂
P.S.: Sorry for a such short blog post, but it’s hard to resume after a pause.